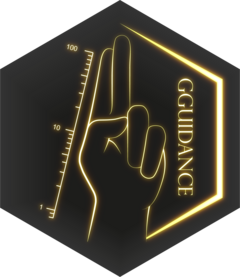
List of theme elements
elements_text.Rd
This is a convenience function for constructing a list of text theme elements. It can take vector input for arguments and pass on the ith value of the vector to the ith call of a function.
Usage
elements_text(
family = NULL,
face = NULL,
colour = NULL,
size = NULL,
hjust = NULL,
vjust = NULL,
angle = NULL,
lineheight = NULL,
color = NULL,
margin = NULL,
debug = NULL,
inherit.blank = FALSE
)
Arguments
- family
Font family
- face
Font face ("plain", "italic", "bold", "bold.italic")
- colour, color
Line/border colour. Color is an alias for colour.
- size
text size in pts.
- hjust
Horizontal justification (in \([0, 1]\))
- vjust
Vertical justification (in \([0, 1]\))
- angle
Angle (in \([0, 360]\))
- lineheight
Line height
- margin
Margins around the text. See
margin()
for more details. When creating a theme, the margins should be placed on the side of the text facing towards the center of the plot.- debug
If
TRUE
, aids visual debugging by drawing a solid rectangle behind the complete text area, and a point where each label is anchored.- inherit.blank
Should this element inherit the existence of an
element_blank
among its parents? IfTRUE
the existence of a blank element among its parents will cause this element to be blank as well. IfFALSE
any blank parent element will be ignored when calculating final element state.
Details
NA
and NULL
values will be silently dropped. If you want to pass
on a transparent colour
argument, it is recommended to use
"transparent"
instead. The dropping of NA
s serve the purpose that you
can skip providing an argument to the text element at the positions where
NA
s occur.
Examples
# Simply have different colours for text
elements_text(colour = c("blue", "red"))
#> [[1]]
#> List of 11
#> $ family : NULL
#> $ face : NULL
#> $ colour : chr "blue"
#> $ size : NULL
#> $ hjust : NULL
#> $ vjust : NULL
#> $ angle : NULL
#> $ lineheight : NULL
#> $ margin : NULL
#> $ debug : NULL
#> $ inherit.blank: logi FALSE
#> - attr(*, "class")= chr [1:2] "element_text" "element"
#>
#> [[2]]
#> List of 11
#> $ family : NULL
#> $ face : NULL
#> $ colour : chr "red"
#> $ size : NULL
#> $ hjust : NULL
#> $ vjust : NULL
#> $ angle : NULL
#> $ lineheight : NULL
#> $ margin : NULL
#> $ debug : NULL
#> $ inherit.blank: logi FALSE
#> - attr(*, "class")= chr [1:2] "element_text" "element"
#>
# More complicated case
elements_text(
# 2nd text will *not* have a family set
family = c("mono", NA, "sans"),
# Arguments that don't take scalars can take lists.
margin = list(NULL, margin(t = 5))
)
#> [[1]]
#> List of 11
#> $ family : chr "mono"
#> $ face : NULL
#> $ colour : NULL
#> $ size : NULL
#> $ hjust : NULL
#> $ vjust : NULL
#> $ angle : NULL
#> $ lineheight : NULL
#> $ margin : NULL
#> $ debug : NULL
#> $ inherit.blank: logi FALSE
#> - attr(*, "class")= chr [1:2] "element_text" "element"
#>
#> [[2]]
#> List of 11
#> $ family : NULL
#> $ face : NULL
#> $ colour : NULL
#> $ size : NULL
#> $ hjust : NULL
#> $ vjust : NULL
#> $ angle : NULL
#> $ lineheight : NULL
#> $ margin : 'margin' num [1:4] 5points 0points 0points 0points
#> ..- attr(*, "unit")= int 8
#> $ debug : NULL
#> $ inherit.blank: logi FALSE
#> - attr(*, "class")= chr [1:2] "element_text" "element"
#>
#> [[3]]
#> List of 11
#> $ family : chr "sans"
#> $ face : NULL
#> $ colour : NULL
#> $ size : NULL
#> $ hjust : NULL
#> $ vjust : NULL
#> $ angle : NULL
#> $ lineheight : NULL
#> $ margin : NULL
#> $ debug : NULL
#> $ inherit.blank: logi FALSE
#> - attr(*, "class")= chr [1:2] "element_text" "element"
#>